某系统需要提供一个命令集合(注:可以使用链表,栈等集合对象实现),用于存储一系列命令对象,并通过该命令集合实现多次undo()和redo()操作,可以使用加法运算来模拟实现。
实验要求:
1. 提交类图;
2. 提交源代码;
3. 注意编程规范。
1、类图
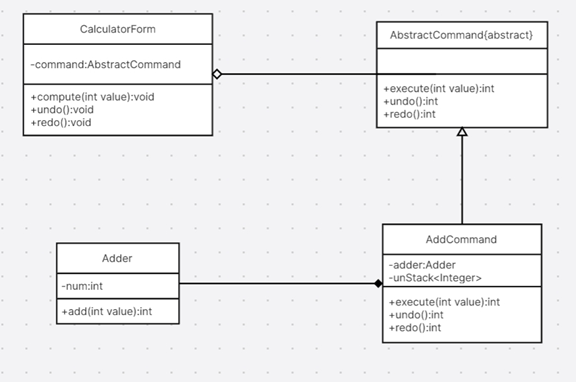
2、源代码
#include<iostream>
#include<stack>
using namespace std;
int num=0;
class AbstractCommand {
public:
virtual int execute(int value)=0;
virtual int undo()=0;
virtual int redo()=0;
};
class Adder {
public:
int add(int value) {
num+=value;
return num;
}
};
class AddCommand :public AbstractCommand {
private:
Adder *adder;
stack<int> unStack;
stack<int> reStack;
public:
int undo() {
//adder= new Adder();
int i=0;
if (unStack.empty()) {
i=-1;
}else{
int pop = unStack.top();
reStack.push(pop);
unStack.pop();
if(!unStack.empty()){//判断弹出数据后是否为空,如果为空,说明已撤回到最原始状态
i=unStack.top();
}
}
return i;
}
int redo() {
//adder= new Adder();
int i=0;
if (reStack.empty()) {
i=-1;
}else{//撤回时只要可以可以撤回,则返回栈一定有数据
// reStack.pop();
int pop = reStack.top();
reStack.pop();
unStack.push(pop);
i=pop;
}
return i;
}
int execute(int value) {
int v = 0;
if (unStack.empty()) {// 说明还没有数据
v = adder->add(value);
unStack.push(v);
} else {// 需要更新两个栈中的内容,并计算结果,其中返回栈应该更新,重复栈应该清空
v = adder->add(value);
unStack.push(v);
if (!reStack.empty()) {
for (int i = 0; i < reStack.size(); i++) {
// reStack.top();
//reStack.pop();
}
}
}
return v;
}
};
class CalculatorForm {
private:
AbstractCommand *command;
public:
void setCommand(AbstractCommand *command) {
this->command =command;
}
void compute(int value) {
command->execute(value);
}
void undo() {
int i = command->undo();
if(i==-1){
cout<<"缓存中已不存在数据"<<endl;
}else{
cout<<"执行成功,运算结果是:"<<i<<endl;
}
}
void redo() {
int i = command->redo();
if(i==-1){
cout<<"已恢复至最新数据"<<endl;
}
else{
cout<<"执行成功,运算结果是:"<<i<<endl;
}
}
};
int main(){
CalculatorForm *form = new CalculatorForm();
AddCommand *command = new AddCommand();
form->setCommand(command);
//计算
cout<<"------计算过程------"<<endl;
form->compute(1);
form->compute(2);
form->compute(3);
form->compute(4);
//多次撤回
cout<<"------撤回过程------"<<endl;
form->undo();
form->undo();
form->undo();
form->undo();
form->undo();
//多次恢复
cout<<"------恢复过程------"<<endl;
form->redo();
form->redo();
form->redo();
form->redo();
form->redo();
return 0;
}