目录
一、删除
(1)在mapper接口执行sql删除语句
① 注解后sql语句没有提示怎么办?
(2)测试层
(3)开启mybatis日志
(4)预编译SQL
二、新增
(1)新增信息
(2)主键返回
三、更新
四、查询
(1)简单查询
当字段名与属性名不一致时,mybatis不封装
① 解决办法1
② 解决办法2
(2)条件查询
五、定义XML映射文件
(1)在resource文件下创建【与mapper接口所在包名一致】的目录文件
(2)在该目录下新建file文件
(3)在xml文件中搭建基础结构
① 获取接口全类名方法
(4)配置sql语句
① 定义方法名后,如何快速在xml文件中生成对应标签?
六、动态SQL
(1)if
(2)foreach
(3)sql&include
一、删除
(1)在mapper接口执行sql删除语句
① 注解后sql语句没有提示怎么办?
@Mapper
public interface EmpMapper {//根据id删除数据@Delete("delete from emp where id = #{id}")public void delete(Integer id);
}
(2)测试层
@SpringBootTest
class MybatisCrudApplicationTests {@Autowiredprivate EmpMapper empmapper;@Testpublic void textDelete() {empmapper.delete(17);}
}
(3)开启mybatis日志
在application.properties配置日志
mybatis.configuration.log-impl=org.apache.ibatis.logging.stdout.StdOutImpl
(4)预编译SQL
更高效:采用?占位符,java发送语句的同时发送参数,后续因为缓存已经有编译好的sql语句,直接可以执行
更安全(防止SQL注入):SQL注入是通过操作输入的数据来修改事先定义好的SQL语句,以达到执行代码对服务器进行攻击的方法
二、新增
(1)新增信息
!注意:#{}内采用【驼峰命名法】,即_用大写字母替代,eg:dept_id → deptId
@Mapper
public interface EmpMapper {//新增员工@Insert("insert into emp (username, name, gender, image, job, entrydate, dept_id, create_time, update_time)" +"values (#{username},#{name},#{gender},#{image},#{job},#{entrydate},#{deptId},#{createTime},#{updateTime})")public void insert(Emp emp);
}
@SpringBootTest
class MybatisCrudApplicationTests {@Autowiredprivate EmpMapper empmapper;@Testpublic void textInsert() {Emp emp = new Emp();emp.setName("tom");emp.setUsername("TOM");emp.setImage("1.jpg");emp.setGender((short)1);emp.setJob((short)1);emp.setEntrydate(LocalDate.of(2000,1,1));emp.setCreateTime(LocalDateTime.now());emp.setUpdateTime(LocalDateTime.now());emp.setId(1);empmapper.insert(emp);}
(2)主键返回
在数据添加成功后,需要获取插入数据的主键。eg:添加套餐数据时,需要返回套餐id来维护套餐-菜品关系
会将自动生成的主键值,赋值给emp对象的id属性
@Options(keyProperty = "id",useGeneratedKeys = true)
//新增员工@Options(keyProperty = "id",useGeneratedKeys = true)@Insert("insert into emp (username, name, gender, image, job, entrydate, dept_id, create_time, update_time)" +"values (#{username},#{name},#{gender},#{image},#{job},#{entrydate},#{deptId},#{createTime},#{updateTime})")public void insert(Emp emp);
三、更新
根据id更新员工信息
@Mapper
public interface EmpMapper {//更新员工@Update("update emp set username = #{username} ,name = #{name},gender = #{gender},image = #{image},job = #{job},entrydate = #{entrydate},dept_id = #{deptId},update_time = #{updateTime} where id = #{id}")public void update(Emp emp);
}
@SpringBootTest
class MybatisCrudApplicationTests {@Autowiredprivate EmpMapper empmapper;@Testpublic void textInsert() {Emp emp = new Emp();emp.setName("kakak");emp.setUsername("88kakk");emp.setImage("1.jpg");emp.setGender((short)1);emp.setJob((short)1);emp.setEntrydate(LocalDate.of(2000,1,1));emp.setUpdateTime(LocalDateTime.now());emp.setId(18);emp.setDeptId(1);empmapper.update(emp);}
}
四、查询
(1)简单查询
@Mapper
public interface EmpMapper {//根据id查询员工@Select("select * from emp where id = #{id}")public Emp getById(Integer id);
}
@SpringBootTest
class MybatisCrudApplicationTests {@Autowiredprivate EmpMapper empmapper;@Testpublic void textInsert() {Emp emp = empmapper.getById(19);System.out.println(emp);}
}
当字段名与属性名不一致时,mybatis不封装
① 解决办法1
手动注解
@Mapper
public interface EmpMapper {//根据id查询员工@Results({@Result(column = "dept_id",property = "deptId"),@Result(column = "crea_time",property = "createTime"),@Result(column = "update_time",property = "updateTime")})@Select("select * from emp where id = #{id}")public Emp getById(Integer id);
}
② 解决办法2
在application.properties开启驼峰命名法开关
# 开启驼峰命名法自动映射开关
mybatis.configuration.map-underscore-to-camel-case=true
(2)条件查询
#{}编译后会被?替代,但?不能出现在‘’内,因此我们不能使用#{},而要使用拼接${},但是${}有sql注入风险,因此我们使用concat()字符串拼接函数
@Mapper
public interface EmpMapper {//根据id查询员工@Select("select * from emp where name like '%${name}%' and gender = #{gender} and entrydate between #{begin} and #{end}")public List<Emp> list(String name, Short gender, LocalDate begin,LocalDate end);
}
@Mapper
public interface EmpMapper {//根据id查询员工@Select("select * from emp where name like concat('%',#{name},'%') and gender = #{gender} and entrydate between #{begin} and #{end}")public List<Emp> list(String name, Short gender, LocalDate begin,LocalDate end);
}
@SpringBootTest
class MybatisCrudApplicationTests {@Autowiredprivate EmpMapper empmapper;@Testpublic void textList() {List<Emp> empList = empmapper.list("张",(short)1,LocalDate.of(2010,1,1),LocalDate.of(2020,1,1));System.out.println(empList);}
}
五、定义XML映射文件
注解开发简单的sql,xml开发动态sql
(1)在resource文件下创建【与mapper接口所在包名一致】的目录文件
(2)在该目录下新建file文件
文件名与mapper接口名一致
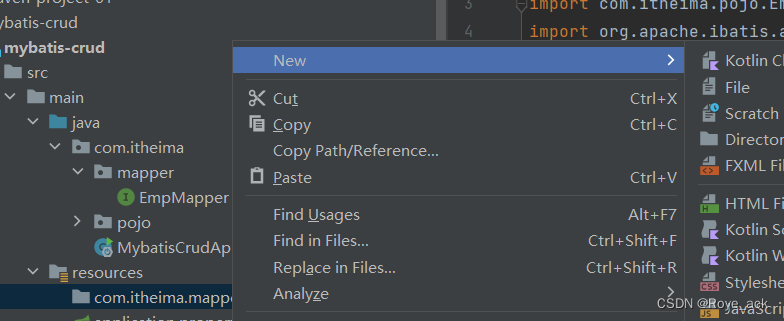
(3)在xml文件中搭建基础结构
namespace属性和mapper接口全类名一致
MyBatis中文网
① 获取接口全类名方法
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapperPUBLIC "-//mybatis.org//DTD Mapper 3.0//EN""http://mybatis.org/dtd/mybatis-3-mapper.dtd"><mapper namespace="com.itheima.mapper.EmpMapper"></mapper>
(4)配置sql语句
id与mapper接口中方法名一致,保持返回类型一致
resultType和类名一致
① 定义方法名后,如何快速在xml文件中生成对应标签?
把光标置于方法名上,按alt+回车+回车,直接跳转到xml文件并生成
六、动态SQL
随着用户的输入或外部条件变化而变化的SQL语句,称为动态SQL
比方说:查询框有姓名,性别,入职时间
用户如果不填某空(缺少某参数),用之前注解sql语句肯定会报错,而动态sql就是解决这一问题的
(1)if
如果test属性成立,则拼接SQL
<mapper namespace="com.itheima.mapper.EmpMapper"><!-- resultType:单条记录所封装的类型--><select id="list" resultType="com.itheima.pojo.Emp">select *from emp<where><if test="name != null">name like concat('%', #{name}, '%')</if><if test="gender != null">and gender = #{gender}</if><if test="begin != null and end != null">and entrydate between #{begin} and #{end}</if></where>order by update_time desc</select></mapper>
(2)foreach
批量删除
- collection:遍历的集合
- item:遍历出来的元素
- separator:分隔符
- open:遍历开始前拼接的SQL片段
- close:遍历结束后拼接的SQL片段
<mapper namespace="com.itheima.mapper.EmpMapper">
<!-- 批量删除员工(18,19,20)--><delete id="deleteByIds">delete from emp where id in<foreach collection="ids" item="x" separator="," open="(" close=")">#{x}</foreach></delete>
</mapper>
@SpringBootTest
class MybatisCrudApplicationTests {@Autowiredprivate EmpMapper empmapper;@Testpublic void textList() {List<Integer> ids = Arrays.asList(13,14,15);empmapper.deleteByIds(ids);}
}
(3)sql&include
sql标签可以择出重复使用的语句,并用include在所需要的地方引/
<mapper namespace="com.itheima.mapper.EmpMapper"><sql id="commonSelect">select id, username, password, name, gender, image, job, entrydate, dept_id, create_time, update_timefrom emp</sql><!-- resultType:单条记录所封装的类型--><select id="list" resultType="com.itheima.pojo.Emp"><include refid="commonSelect"/><where><if test="name != null">name like concat('%', #{name}, '%')</if><if test="gender != null">and gender = #{gender}</if><if test="begin != null and end != null">and entrydate between #{begin} and #{end}</if></where>order by update_time desc</select></mapper>