文章目录
- 一、基本概念
- 二、控制流
- 三、函数
- 四、模块
- 五、数据结构
- 六、面向对象的编程
- 七、输入输出
- 八、异常
- 九、Python标准库
- 关于Python技术储备
- 一、Python所有方向的学习路线
- 二、Python基础学习视频
- 三、精品Python学习书籍
- 四、Python工具包+项目源码合集
- ①Python工具包
- ②Python实战案例
- ③Python小游戏源码
- 五、面试资料
- 六、Python兼职渠道
一、基本概念
1、数
在Python中有4种类型的数——整数、长整数、浮点数和复数。
(1)2是一个整数的例子。
(2)长整数不过是大一些的整数。
(2)3.23和52.3E-4是浮点数的例子。E标记表示10的幂。在这里,52.3E-4表示52.3 * 10-4。
(4)(-5+4j)和(2.3-4.6j)是复数的例子。
2、字符串
(1)使用单引号(‘)
(2)使用双引号(")
(3)使用三引号(’''或"“”)
利用三引号,你可以指示一个多行的字符串。你可以在三引号中自由的使用单引号和双引号。例如:
'''This is a multi-line string. This is the first line.
This is the second line.
"What's your name?," I asked.
He said "Bond, James Bond."
'''
(4)转义符
(5)自然字符串
自然字符串通过给字符串加上前缀r或R来指定。例如r"Newlines are indicated by \n"。
3、逻辑行与物理行
一个物理行中使用多于一个逻辑行,需要使用分号(;)来特别地标明这种用法。一个物理行只有一个逻辑行可不用分号
二、控制流
1、if
块中不用大括号,条件后用分号,对应elif和else
if guess == number:print 'Congratulations, you guessed it.' # New block starts here
elif guess < number:print 'No, it is a little higher than that' # Another block
else:print 'No, it is a little lower than that'
2、while
用分号,可搭配else
while running:guess = int(raw\_input('Enter an integer : '))if guess == number:print 'Congratulations, you guessed it.'running = False # this causes the while loop to stopelif guess < number:print 'No, it is a little higher than that'else:print 'No, it is a little lower than that'
else:print 'The while loop is over.'# Do anything else you want to do here
3、for
用分号,搭配else
for i in range(1, 5):print i
else:print 'The for loop is over'
4、break和continue
同C语言
三、函数
1、定义与调用
def sayHello():print 'Hello World!' # block belonging to the function
sayHello() # call the function
2、函数形参
类C语言
def printMax(a, b):if a > b:print a, 'is maximum'else:print b, 'is maximum'
3、局部变量
加global可申明为全局变量
4、默认参数值
def say(message, times = 1):print message \* times
5、关键参数
如果某个函数有许多参数,而只想指定其中的一部分,那么可以通过命名来为这些参数赋值——这被称作 关键参数 ——使用名字(关键字)而不是位置来给函数指定实参。这样做有两个 优势 ——一,由于不必担心参数的顺序,使用函数变得更加简单了。二、假设其他参数都有默认值,可以只给我们想要的那些参数赋值。
def func(a, b=5, c=10):print 'a is', a, 'and b is', b, 'and c is', c
func(3, 7)
func(25, c=24)
func(c=50, a=100)
6、return
四、模块
1、使用模块
import sys
print 'The command line arguments are:'
for i in sys.argv:print i
如果想要直接输入argv变量到程序中(避免在每次使用它时打sys.),可以使用from sys import argv语句
2、dir()函数
可以使用内建的dir函数来列出模块定义的标识符。标识符有函数、类和变量。
五、数据结构
1、列表
shoplist = \['apple', 'mango', 'carrot', 'banana'\]
print 'I have', len(shoplist),'items to purchase.'
print 'These items are:', # Notice the comma at end of the line
for item in shoplist:print item,
print '\\nI also have to buy rice.'
shoplist.append('rice')
print 'My shopping list is now', shoplist
print 'I will sort my list now'
shoplist.sort()
print 'Sorted shopping list is', shoplist
print 'The first item I will buy is', shoplist\[0\]
olditem = shoplist\[0\]
del shoplist\[0\]
print 'I bought the', olditem
print 'My shopping list is now', shoplist
2、元组
元组和列表十分类似,只不过元组和字符串一样是不可变的即你不能修改元组。
zoo = ('wolf', 'elephant', 'penguin')
print 'Number of animals in the zoo is', len(zoo)
new\_zoo = ('monkey', 'dolphin', zoo)
print 'Number of animals in the new zoo is', len(new\_zoo)
print 'All animals in new zoo are', new\_zoo
print 'Animals brought from old zoo are', new\_zoo\[2\]
print 'Last animal brought from old zoo is', new\_zoo\[2\]\[2\]
像一棵树
元组与打印
age = 22
name = 'Swaroop'
print '%s is %d years old' % (name, age)
print 'Why is %s playing with that python?' % name
3、字典
类似哈希
ab = { 'Swaroop' : 'swaroopch@byteofpython.info','Larry' : 'larry@wall.org','Matsumoto' : 'matz@ruby-lang.org','Spammer' : 'spammer@hotmail.com'}
print "Swaroop's address is %s" % ab\['Swaroop'\]
# Adding a key/value pair
ab\['Guido'\] = 'guido@python.org'
# Deleting a key/value pair
del ab\['Spammer'\]
print '\\nThere are %d contacts in the address-book\\n' % len(ab)
for name, address in ab.items():print 'Contact %s at %s' % (name, address)
if 'Guido' in ab: # OR ab.has\_key('Guido')print "\\nGuido's address is %s" % ab\['Guido'\]
4、序列
列表、元组和字符串都是序列。序列的两个主要特点是索引操作符和切片操作符。
shoplist = \['apple', 'mango', 'carrot', 'banana'\]
# Indexing or 'Subscription' operation
print 'Item 0 is', shoplist\[0\]
print 'Item 1 is', shoplist\[1\]
print 'Item -1 is', shoplist\[-1\]
print 'Item -2 is', shoplist\[-2\]
# Slicing on a list
print 'Item 1 to 3 is', shoplist\[1:3\]
print 'Item 2 to end is', shoplist\[2:\]
print 'Item 1 to -1 is', shoplist\[1:-1\]
print 'Item start to end is', shoplist\[:\]
# Slicing on a string
name = 'swaroop'
print 'characters 1 to 3 is', name\[1:3\]
print 'characters 2 to end is', name\[2:\]
print 'characters 1 to -1 is', name\[1:-1\]
print 'characters start to end is', name\[:\]
5、参考
当你创建一个对象并给它赋一个变量的时候,这个变量仅仅参考那个对象,而不是表示这个对象本身!也就是说,变量名指向你计算机中存储那个对象的内存。这被称作名称到对象的绑定。
print 'Simple Assignment'
shoplist = \['apple', 'mango', 'carrot', 'banana'\]
mylist = shoplist # mylist is just another name pointing to the same object!
del shoplist\[0\]
print 'shoplist is', shoplist
print 'mylist is', mylist
# notice that both shoplist and mylist both print the same list without
# the 'apple' confirming that they point to the same object
print 'Copy by making a full slice'
mylist = shoplist\[:\] # make a copy by doing a full slice
del mylist\[0\] # remove first item
print 'shoplist is', shoplist
print 'mylist is', mylist
# notice that now the two lists are different
6、字符串
name = 'Swaroop' # This is a string object
if name.startswith('Swa'):print 'Yes, the string starts with "Swa"'
if 'a' in name:print 'Yes, it contains the string "a"'
if name.find('war') != -1:print 'Yes, it contains the string "war"'
delimiter = '\_\*\_'
mylist = \['Brazil', 'Russia', 'India', 'China'\]
print delimiter.join(mylist) //用delimiter来连接mylist的字符
六、面向对象的编程
1、self
Python中的self等价于C++中的self指针和Java、C#中的this参考
2、创建类
class Person:pass # An empty block
p = Person()
print p
3、对象的方法
class Person:def sayHi(self):print 'Hello, how are you?'
p = Person()
p.sayHi()
4、初始化
class Person:def \_\_init\_\_(self, name):self.name = namedef sayHi(self):print 'Hello, my name is', self.name
p = Person('Swaroop')
p.sayHi()
5、类与对象的方法
类的变量 由一个类的所有对象(实例)共享使用。只有一个类变量的拷贝,所以当某个对象对类的变量做了改动的时候,这个改动会反映到所有其他的实例上。
对象的变量 由类的每个对象/实例拥有。因此每个对象有自己对这个域的一份拷贝,即它们不是共享的,在同一个类的不同实例中,虽然对象的变量有相同的名称,但是是互不相关的。
class Person:'''Represents a person.'''population = 0def \_\_init\_\_(self, name):'''Initializes the person's data.'''self.name = nameprint '(Initializing %s)' % self.name# When this person is created, he/she# adds to the populationPerson.population += 1
population属于Person类,因此是一个类的变量。name变量属于对象(它使用self赋值)因此是对象的变量。
6、继承
class SchoolMember:'''Represents any school member.'''def \_\_init\_\_(self, name, age):self.name = name
class Teacher(SchoolMember):'''Represents a teacher.'''def \_\_init\_\_(self, name, age, salary):SchoolMember.\_\_init\_\_(self, name, age)self.salary = salary
七、输入输出
1、文件
f = file('poem.txt', 'w') # open for 'w'riting
f.write(poem) # write text to file
f.close() # close the file
f = file('poem.txt')
# if no mode is specified, 'r'ead mode is assumed by default
while True:line = f.readline()if len(line) == 0: # Zero length indicates EOFbreakprint line,# Notice comma to avoid automatic newline added by Python
f.close() # close the file
2、存储器
持久性
import cPickle as p
#import pickle as p
shoplistfile = 'shoplist.data'
# the name of the file where we will store the object
shoplist = \['apple', 'mango', 'carrot'\]
# Write to the file
f = file(shoplistfile, 'w')
p.dump(shoplist, f) # dump the object to a file
f.close()
del shoplist # remove the shoplist
# Read back from the storage
f = file(shoplistfile)
storedlist = p.load(f)
print storedlist
3、控制台输入
输入字符串 nID = raw_input(“Input your id plz”)
输入整数 nAge = int(raw_input(“input your age plz:\n”))
输入浮点型 fWeight = float(raw_input(“input your weight\n”))
输入16进制数据 nHex = int(raw_input(‘input hex value(like 0x20):\n’),16)
输入8进制数据 nOct = int(raw_input(‘input oct value(like 020):\n’),8)
八、异常
1、try…except
import sys
try:s = raw\_input('Enter something --> ')
except EOFError:print '\\nWhy did you do an EOF on me?'sys.exit() # exit the program
except:print '\\nSome error/exception occurred.'# here, we are not exiting the program
print 'Done'
2、引发异常
使用raise语句引发异常。你还得指明错误/异常的名称和伴随异常 触发的 异常对象。你可以引发的错误或异常应该分别是一个Error或Exception类的直接或间接导出类。
class ShortInputException(Exception):'''A user-defined exception class.'''def \_\_init\_\_(self, length, atleast):Exception.\_\_init\_\_(self)self.length = lengthself.atleast = atleast
raise ShortInputException(len(s), 3)
3、try…finnally
import time
try:f = file('poem.txt')while True: # our usual file-reading idiomline = f.readline()if len(line) == 0:breaktime.sleep(2)print line,
finally:f.close()print 'Cleaning up...closed the file'
九、Python标准库
1、sys库
sys模块包含系统对应的功能。sys.argv列表,它包含命令行参数。
2、os库
os.name字符串指示你正在使用的平台。比如对于Windows,它是’nt’,而对于Linux/Unix用户,它是’posix’。
os.getcwd()函数得到当前工作目录,即当前Python脚本工作的目录路径。
os.getenv()和os.putenv()函数分别用来读取和设置环境变量。
os.listdir()返回指定目录下的所有文件和目录名。
os.remove()函数用来删除一个文件。
os.system()函数用来运行shell命令。
os.linesep字符串给出当前平台使用的行终止符。例如,Windows使用’\r\n’,Linux使用’\n’而Mac使用’\r’。
os.path.split()函数返回一个路径的目录名和文件名。
>>> os.path.split(‘/home/swaroop/byte/code/poem.txt’)
(‘/home/swaroop/byte/code’, ‘poem.txt’)
os.path.isfile()和os.path.isdir()函数分别检验给出的路径是一个文件还是目录。类似地,os.path.existe()函数用来检验给出的路径是否真地存在。
关于Python技术储备
学好 Python 不论是就业还是做副业赚钱都不错,但要学会 Python 还是要有一个学习规划。最后大家分享一份全套的 Python 学习资料,给那些想学习 Python 的小伙伴们一点帮助!
微信扫描下方CSDN官方认证二维码免费领取【保证100%免费
】
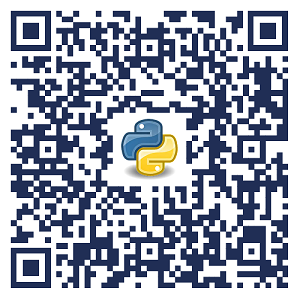
一、Python所有方向的学习路线
Python所有方向的技术点做的整理,形成各个领域的知识点汇总,它的用处就在于,你可以按照上面的知识点去找对应的学习资源,保证自己学得较为全面。
二、Python基础学习视频
② 路线对应学习视频
还有很多适合0基础入门的学习视频,有了这些视频,轻轻松松上手Python~在这里插入图片描述
③练习题
每节视频课后,都有对应的练习题哦,可以检验学习成果哈哈!
因篇幅有限,仅展示部分资料
三、精品Python学习书籍
当我学到一定基础,有自己的理解能力的时候,会去阅读一些前辈整理的书籍或者手写的笔记资料,这些笔记详细记载了他们对一些技术点的理解,这些理解是比较独到,可以学到不一样的思路。
四、Python工具包+项目源码合集
①Python工具包
学习Python常用的开发软件都在这里了!每个都有详细的安装教程,保证你可以安装成功哦!
②Python实战案例
光学理论是没用的,要学会跟着一起敲代码,动手实操,才能将自己的所学运用到实际当中去,这时候可以搞点实战案例来学习。100+实战案例源码等你来拿!
③Python小游戏源码
如果觉得上面的实战案例有点枯燥,可以试试自己用Python编写小游戏,让你的学习过程中增添一点趣味!
五、面试资料
我们学习Python必然是为了找到高薪的工作,下面这些面试题是来自阿里、腾讯、字节等一线互联网大厂最新的面试资料,并且有阿里大佬给出了权威的解答,刷完这一套面试资料相信大家都能找到满意的工作。
六、Python兼职渠道
而且学会Python以后,还可以在各大兼职平台接单赚钱,各种兼职渠道+兼职注意事项+如何和客户沟通,我都整理成文档了。
这份完整版的Python全套学习资料已经上传CSDN,朋友们如果需要可以微信扫描下方CSDN官方认证二维码免费领取【保证100%免费
】
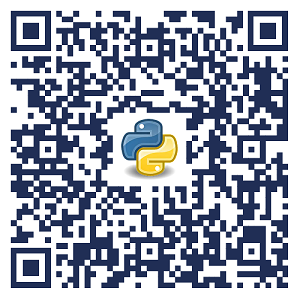