Vue3+java开发组队功能
Vue3+java开发系统组队功能
需求分析
- 创建用户可以创建一个队伍(一个房间队长),设置队伍人数,队伍名称(标题),描述,超时时间。
- 搜索
- 加入,用户可以加入未满的队伍(其他人,未满,未过期),是否需要队长同意
- 分享队伍,邀请人员
- 显示队伍人数
- 聊天
- 修改队伍信息
- 退出
- 解散
系统(接口)设计
- 判断请求参数是否为空
- 是否登录,未登录直接跳转到登录,不允许创建
- 校验信息
- 队伍人数大于1小于20
- 队伍名称<=20
- 队伍人数<=412
- 是否公开(int)不穿默认位0,公开
- 如果是加密状态,一定3要有密码,且密码<=32
- 超时时间>当前时间
- 校验用户最多创建5个队伍
- 插入队伍信息到队伍表
- 插入用户 => 队伍关系到关系表
实现
1. 库表设计(10min)
- 数据库表设计,队伍表,队伍用户表
create table team
(id bigint auto_increment comment 'id'primary key,name varchar(256) not null comment '队伍名称',description varchar(1024) null comment '描述',maxNum int default 1 not null comment '最大人数',expireTime datetime null comment '过期时间',userId bigint comment '用户id',status int default 0 not null comment '0 - 公开,1 - 私有,2 - 加密',password varchar(512) null comment '密码',createTime datetime default CURRENT_TIMESTAMP null comment '创建时间',updateTime datetime default CURRENT_TIMESTAMP null on update CURRENT_TIMESTAMP,isDelete tinyint default 0 not null comment '是否删除'
)comment '队伍';
create table user_team
(id bigint auto_increment comment 'id'primary key,userId bigint comment '用户id',teamId bigint comment '队伍id',joinTime datetime null comment '加入时间',createTime datetime default CURRENT_TIMESTAMP null comment '创建时间',updateTime datetime default CURRENT_TIMESTAMP null on update CURRENT_TIMESTAMP,isDelete tinyint default 0 not null comment '是否删除'
)comment '用户队伍关系';
2. 增删改查代码实现(10min)
- 使用mybatisX-generation插件自动生成实体类服务层,持久层代码
- 队伍基本增删改查代码编写
@RestController
@RequestMapping("/team")
@CrossOrigin(origins = {"http://localhost:5173"}, allowCredentials = "true")
@Slf4j
public class TeamController {@Resourceprivate UserService userService;@Resourceprivate TeamService teamService;@PostMapping("/add")public BaseResponse<Long> addTeam(@RequestBody Team team){if(team == null){throw new BusinessException(ErrorCode.PARAMS_ERROR);}boolean save = teamService.save(team);if(!save){throw new BusinessException(ErrorCode.SYSTEM_ERROR,"插入失败");}return ResultUtils.success(team.getId());}@PostMapping("/delete")public BaseResponse<Boolean> deleteTeam(@RequestBody long id){if(id <= 0){throw new BusinessException(ErrorCode.PARAMS_ERROR);}boolean result = teamService.removeById(id);if(!result){throw new BusinessException(ErrorCode.SYSTEM_ERROR,"删除失败");}return ResultUtils.success(true);}@PostMapping("/delete")public BaseResponse<Boolean> updateTeam(@RequestBody Team team){if(team == null){throw new BusinessException(ErrorCode.PARAMS_ERROR);}boolean result = teamService.updateById(team);if(!result){throw new BusinessException(ErrorCode.SYSTEM_ERROR,"更新失败");}return ResultUtils.success(true);}@GetMapping("/delete")public BaseResponse<Team> getTeamById(@RequestBody long id){if(id <= 0){throw new BusinessException(ErrorCode.PARAMS_ERROR);}Team team = teamService.getById(id);if(team == null){throw new BusinessException(ErrorCode.NULL_ERROR,"数据为空!");}return ResultUtils.success(team);}
}
- 查询队伍列表功能实现
- 新建TeamQuery业务请求参数封装类作为作为参数
- 实现查询队伍列表
@GetMapping("/list")
public BaseResponse<List<Team>> listTeams(TeamQuery teamQuery){if (teamQuery == null){throw new BusinessException(ErrorCode.PARAMS_ERROR);}Team team = new Team();BeanUtils.copyProperties(team,teamQuery);QueryWrapper<Team> queryWrapper = new QueryWrapper<>();List<Team> teamList = teamService.list(queryWrapper);return ResultUtils.success(teamList);
}
- 分页查询队伍列表功能实现
- 新建请求分页类
@Data
public class PageRequest implements Serializable {private static final long serialVersionUID = -9075033996918167511L;protected int pageSize;protected int pageNum;
}
- 分页查询队伍实现代码
@GetMapping("/list/page")
public BaseResponse<Page<Team>> listTeamsByPage(TeamQuery teamQuery){if(teamQuery == null){throw new BusinessException(ErrorCode.PARAMS_ERROR);}Team team = new Team();BeanUtils.copyProperties(team, teamQuery);Page<Team> page = new Page<>(teamQuery.getPageNum(), teamQuery.getPageSize());QueryWrapper<Team> queryWrapper = new QueryWrapper<>(team);Page<Team> Resultpage = teamService.page(page, queryWrapper);return ResultUtils.success(Resultpage);}
- 使用Swagger+knif4j文档接口
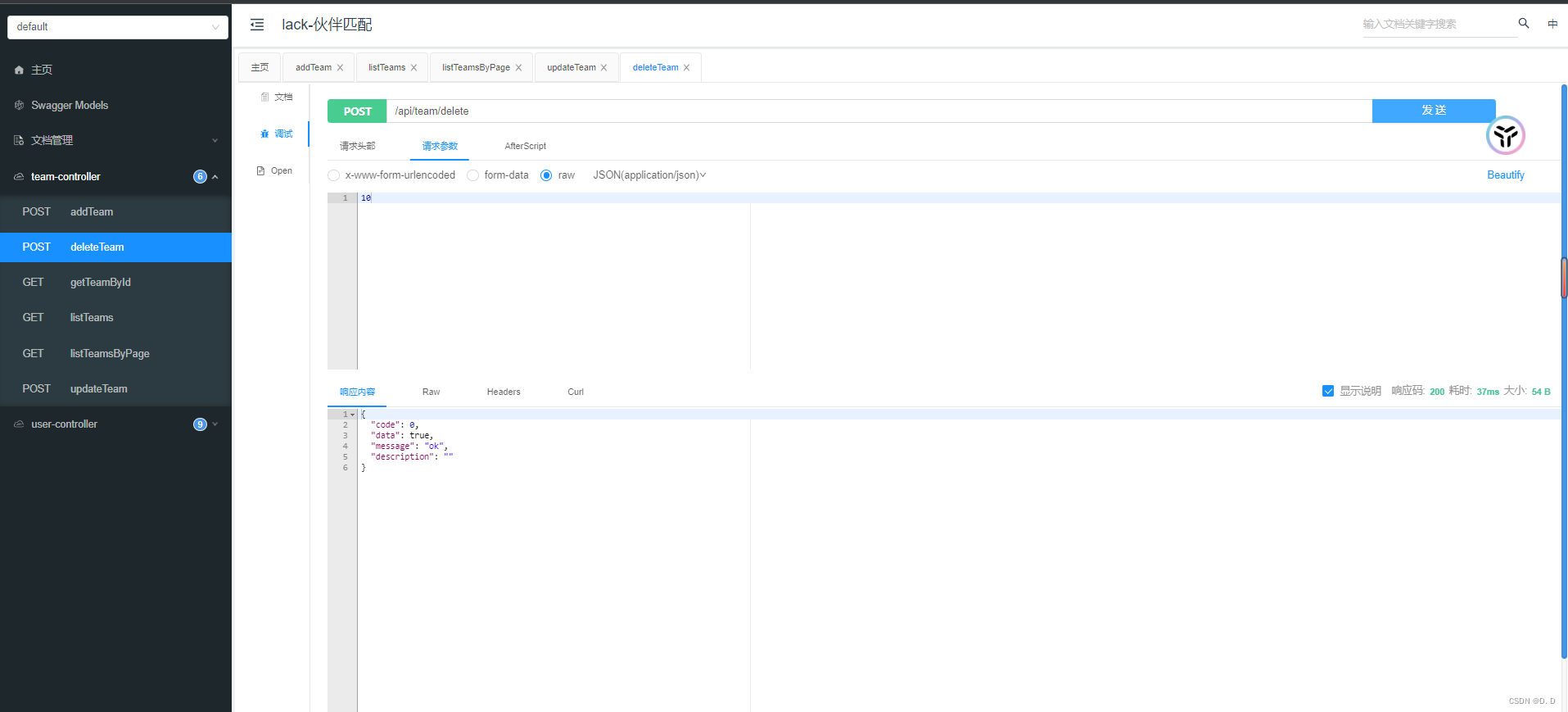
3. 业务逻辑(30min)
- 创建队伍业务逻辑实现
@Service
public class TeamServiceImpl extends ServiceImpl<TeamMapper, Team>implements TeamService {@Resourceprivate UserTeamService userTeamService;@Override@Transactional(rollbackFor = Exception.class)public long addTeam(Team team, User loginUser) {if (team == null) {throw new BusinessException(ErrorCode.PARAMS_ERROR);}if (loginUser == null) {throw new BusinessException(ErrorCode.NO_AUTH);}final long userId = loginUser.getId();int maxNum = Optional.ofNullable(team.getMaxNum()).orElse(0);if (maxNum < 1 || maxNum > 20) {throw new BusinessException(ErrorCode.PARAMS_ERROR, "队伍人数不满足要求");}String name = team.getName();if (StringUtils.isBlank(name) || name.length() > 20) {throw new BusinessException(ErrorCode.PARAMS_ERROR, "队伍标题不满足要求");}String description = team.getDescription();if (StringUtils.isNotBlank(description) && description.length() > 512) {throw new BusinessException(ErrorCode.PARAMS_ERROR, "队伍描述过长");}int status = Optional.ofNullable(team.getStatus()).orElse(0);TeamStatusEnum statusEnum = TeamStatusEnum.getEnumByValue(status);if (statusEnum == null) {throw new BusinessException(ErrorCode.PARAMS_ERROR, "队伍状态不满足要求");}String password = team.getPassword();if (TeamStatusEnum.SECRET.equals(statusEnum)) {if (StringUtils.isBlank(password) || password.length() > 32) {throw new BusinessException(ErrorCode.PARAMS_ERROR, "密码设置不正确");}}Date expireTime = team.getExpireTime();if (new Date().after(expireTime)) {throw new BusinessException(ErrorCode.PARAMS_ERROR, "超出时间 > 当前时间");}QueryWrapper<Team> queryWrapper = new QueryWrapper<>();queryWrapper.eq("userId", userId);long hasTeamNum = this.count(queryWrapper);if (hasTeamNum >= 5) {throw new BusinessException(ErrorCode.PARAMS_ERROR, "用户最多创建5个队伍");}team.setId(null);team.setUserId(userId);boolean result = this.save(team);Long teamId = team.getId();if (!result || teamId == null) {throw new BusinessException(ErrorCode.PARAMS_ERROR, "创建队伍失败");}UserTeam userTeam = new UserTeam();userTeam.setUserId(userId);userTeam.setTeamId(teamId);userTeam.setJoinTime(new Date());result = userTeamService.save(userTeam);if (!result) {throw new BusinessException(ErrorCode.PARAMS_ERROR, "创建队伍失败");}return teamId;}
}
本文来自互联网用户投稿,该文观点仅代表作者本人,不代表本站立场。本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。如若转载,请注明出处:http://www.hqwc.cn/news/229377.html
如若内容造成侵权/违法违规/事实不符,请联系编程知识网进行投诉反馈email:809451989@qq.com,一经查实,立即删除!