1.核心配置文件SqlMapConfig.xml
1.1 MyBatis核心配置文件层级关系
1.2MyBatis常用配置解析
1)environments标签
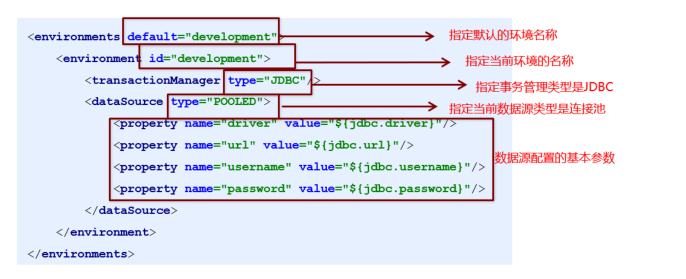
其中,事务管理器(transactionManager)类型有两种:
- JDBC:这个配置就是直接使用了JDBC 的提交和回滚设置,它依赖于从数据源得到的连接来管理事务作用域。
- MANAGED:这个配置几乎没做什么。它从来不提交或回滚一个连接,而是让容器来管理事务的整个生命周期(比如 JEE 应用服务器的上下文)。 默认情况下它会关闭连接,然而一些容器并不希望这样,因此需要将
closeConnection 属性设置为 false 来阻止它默认的关闭行为
其中,数据源(dataSource)类型有三种:
- UNPOOLED:这个数据源的实现只是每次被请求时打开和关闭连接。
- POOLED:这种数据源的实现利用“池”的概念将 JDBC 连接对象组织起来。
- JNDI:这个数据源的实现是为了能在如 EJB 或应用服务器这类容器中使用,容器可以集中或在外部配置数据源,然后放置一个 JNDI 上下文的引用。
2)mapper标签
该标签的作用是加载映射的,加载方式有如下几种:
//使用相对于类路径的资源引用,例如:
<mapper resource="org/mybatis/builder/AuthorMapper.xml"/>
//使用完全限定资源定位符(URL),例如:
<mapper url="file:///var/mappers/AuthorMapper.xml"/>
//使用映射器接口实现类的完全限定类名,例如:
<mapper class="org.mybatis.builder.AuthorMapper"/>
//将包内的映射器接口实现全部注册为映射器,要求和mapper同包同名 例如:
<package name="org.mybatis.builder"/>
3)Properties标签
实际开发中,习惯将数据源的配置信息单独抽取成一个properties文件,该标签可以加载额外配置的properties文件
jdbc.properties
jdbc.driver=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql:///test
jdbc.username=root
jdbc.password=123456
SqlMapConfig.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE configurationPUBLIC "-//mybatis.org//DTD Config 3.0//EN""http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration><!--加载外部properties--><properties resource="jdbc.properties"></properties><environments default="development"><environment id="development"><!--当前事务交给JDBC处理--><transactionManager type="JDBC"/><!--当前使用mybatis提供的连接池--><dataSource type="POOLED"><property name="driver" value="com.mysql.jdbc.Driver"/><property name="url" value="jdbc:mysql:///test"/><property name="username" value="root"/><property name="password" value="123456"/></dataSource></environment></environments><!--引入映射配置文件--><mappers><mapper resource="UserMapper.xml"/><mapper resource="UserMapperProxy.xml"/></mappers>
</configuration>
4)typeAliases标签
类型别名是为Java 类型设置一个短的名字。原来的类型名称配置如下 **
**
配置typeAliases,为com.cookie.pojo.User定义别名为user
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE configurationPUBLIC "-//mybatis.org//DTD Config 3.0//EN""http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration><!--加载外部properties--><properties resource="jdbc.properties"></properties><!--给实体类的全限定类名起别名--><typeAliases><typeAlias type="com.cookie.pojo.User" alias="user"></typeAlias></typeAliases><environments default="development"><environment id="development"><!--当前事务交给JDBC处理--><transactionManager type="JDBC"/><!--当前使用mybatis提供的连接池--><dataSource type="POOLED"><property name="driver" value="com.mysql.jdbc.Driver"/><property name="url" value="jdbc:mysql:///test"/><property name="username" value="root"/><property name="password" value="123456"/></dataSource></environment></environments><!--引入映射配置文件--><mappers><mapper resource="UserMapper.xml"/><mapper resource="UserMapperProxy.xml"/></mappers>
</configuration>
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapperPUBLIC "-//mybatis.org//DTD Mapper 3.0//EN""http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.cookie.mapper.UserMapper"><!--namespace :命名空间:与id组成sql的唯一标识--><!--resultType:表明返回值类型--><select id="findAll" resultType="user">select * from user</select><!--添加--><insert id="add" parameterType="user">insert into user values(#{id},#{username},#{password})</insert><!--修改--><update id="update" parameterType="user">update user set username = #{username},password = #{password} where id = #{id}</update><!--删除--><delete id="delete" parameterType="java.lang.Integer">delete from user where id = #{id}</delete></mapper>
<!--给实体类的全限定类名起别名--><typeAliases><!--给单独的实体起别名--><typeAlias type="com.cookie.pojo.User" alias="user"></typeAlias><!--给包下所有类起别名 不区分大小写--><package name="com.cookie.pojo"/></typeAliases>
上面是自定义的别名, mybatis框架已经设置好的一些常用的类型的别名:
2.映射配置文件mapper.xml
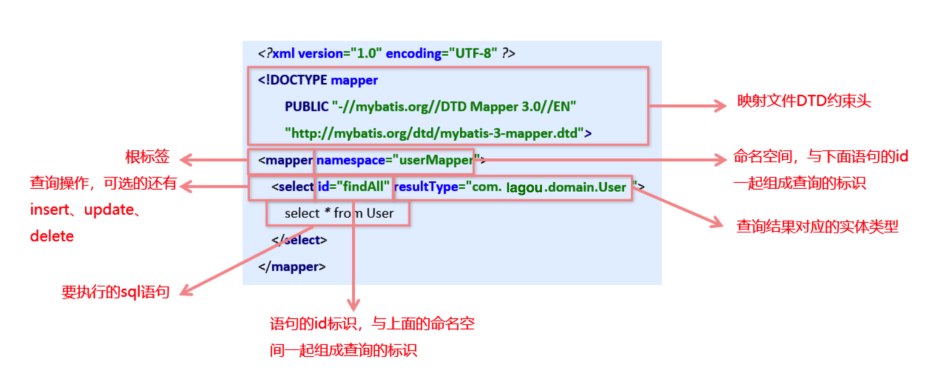
动态sql语句概述:
Mybatis 的映射文件中,前面的 SQL 都是比较简单的,有些时候业务逻辑复杂时,SQL是动态变化
的,此时前面的 SQL 就不能满足要求了。
官方文档,描述如下:
动态 SQL 之
根据实体类的不同取值,使用不同的 SQL语句来进行查询。比如在 id如果不为空时可以根据id查询,如果username 不同空时还要加入用户名作为条件。这种情况在多条件组合查询中经常会碰到。
<select id="findByCondition" parameterType="user" resultType="user">select * from user where 1=1<if test="id != 3">and id = #{id}</if><if test="username != null">and username = #{username}</if></select>
能行但是不太好,用标签
<select id="findByCondition" parameterType="user" resultType="user">select * from user<where><if test="id != 3">and id = #{id}</if><if test="username != null">and username = #{username}</if></where></select>
public interface UserMapper {List<User> findAll();List<User> findByCondition(User user);
}
@Testpublic void testProxy2() throws IOException {InputStream resourceAsStream = Resources.getResourceAsStream("SqlMapConfig.xml");SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(resourceAsStream);SqlSession sqlSession = sqlSessionFactory.openSession();UserMapper mapper = sqlSession.getMapper(UserMapper.class);User newUser = new User();newUser.setId(3);newUser.setUsername("cookie");List<User> users = mapper.findByCondition(newUser);for (User user : users) {System.out.println(user);}}
动态 SQL 之
循环执行sql的拼接操作,例如: SELECT * FROM USER WHERE id IN (1,2,5)。
<select id="findByIds" parameterType="list" resultType="user">select * from user<where><foreach collection="list" open="id in (" close=")" item="id" separator=",">#{id}</foreach></where></select>
public interface UserMapper {List<User> findAll();List<User> findByCondition(User user);List<User> findByIds(List<Integer> list);
}
@Testpublic void testProxy3() throws IOException {InputStream resourceAsStream = Resources.getResourceAsStream("SqlMapConfig.xml");SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(resourceAsStream);SqlSession sqlSession = sqlSessionFactory.openSession();UserMapper mapper = sqlSession.getMapper(UserMapper.class);List<Integer> list = new ArrayList<>();list.add(3);list.add(2);List<User> users = mapper.findByIds(list);for (User user : users) {System.out.println(user);}}
foreach标签的属性含义如下:
标签用于遍历集合,它的属性:
- collection:代表要遍历的集合元素,注意编写时不要写#{}
- open:代表语句的开始部分
- close:代表结束部分
- item:代表遍历集合的每个元素,生成的变量名
- sperator:代表分隔符
SQL片段抽取
Sql 中可将重复的 sql 提取出来,使用时用 include 引用即可,最终达到 sql 重用的目的
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapperPUBLIC "-//mybatis.org//DTD Mapper 3.0//EN""http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.cookie.mapper.UserMapper"><!--namespace :命名空间:与id组成sql的唯一标识--><!--resultType:表明返回值类型--><!--抽取sql片段简化编写--><sql id="selectUser">select * from user</sql><select id="findAll" resultType="user"><include refid="selectUser"></include></select><!--添加--><insert id="add" parameterType="user">insert into user values(#{id},#{username},#{password})</insert><!--修改--><update id="update" parameterType="user">update user set username = #{username},password = #{password} where id = #{id}</update><!--删除--><delete id="delete" parameterType="java.lang.Integer">delete from user where id = #{id}</delete><select id="findByCondition" parameterType="user" resultType="user"><include refid="selectUser"></include><where><if test="id != 3">and id = #{id}</if><if test="username != null">and username = #{username}</if></where></select><select id="findByIds" parameterType="list" resultType="user"><include refid="selectUser"></include><where><foreach collection="list" open="id in (" close=")" item="id" separator=",">#{id}</foreach></where></select></mapper>