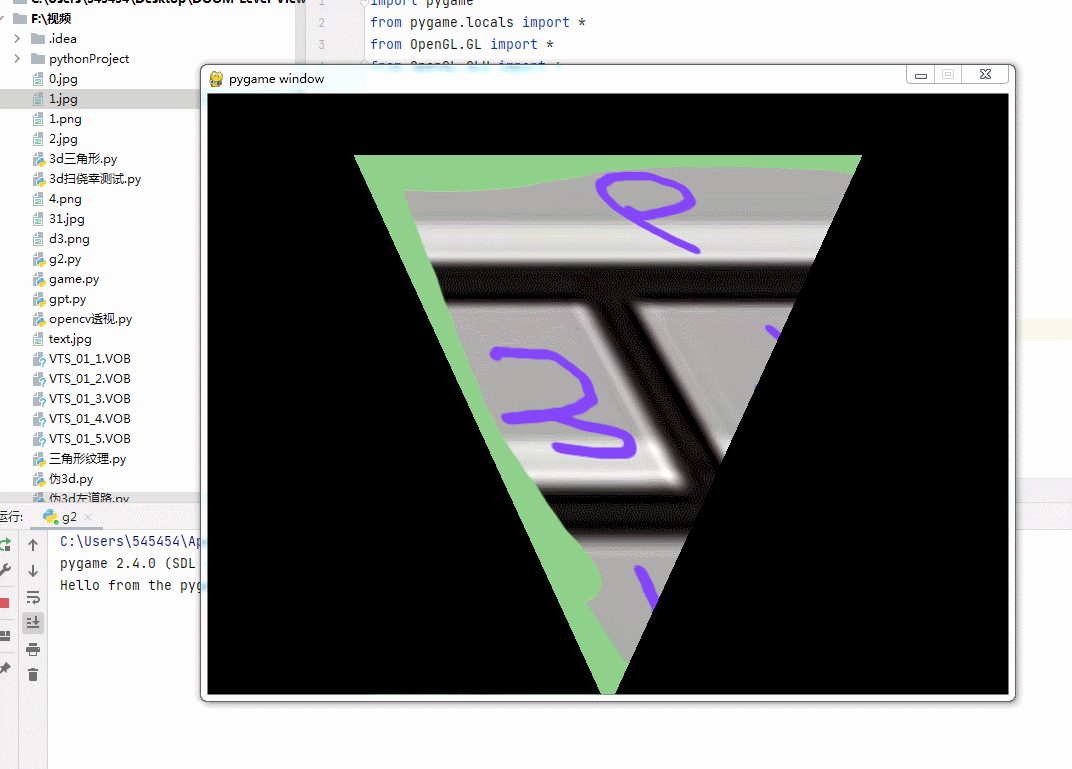
import pygame
from pygame.locals import *
from OpenGL.GL import *
from OpenGL.GLU import *def draw_triangle():vertices = ((0, 2, 0), (-2, -2, 0), (2, -2, 0) )tex_coords = ((1, 2), (1, 1), (2, 1) )texture_surface = pygame.image.load('1.jpg')texture_data = pygame.image.tostring(texture_surface, 'RGB', 1)width = texture_surface.get_width()height = texture_surface.get_height()texture_id = glGenTextures(1)glBindTexture(GL_TEXTURE_2D, texture_id)glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR)glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR)glTexImage2D(GL_TEXTURE_2D, 0, GL_RGB, width, height, 0, GL_RGB, GL_UNSIGNED_BYTE, texture_data)glBegin(GL_TRIANGLES)for i in range(len(vertices)):glTexCoord2fv(tex_coords[i])glVertex3fv(vertices[i])glEnd()def main():pygame.init()display = (800, 600)pygame.display.set_mode(display, DOUBLEBUF | OPENGL)gluPerspective(45, (display[0] / display[1]), 0.1, 50.0)glTranslatef(0.0, 0.0, -5)while True:for event in pygame.event.get():if event.type == pygame.QUIT:pygame.quit()quit()glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT)glEnable(GL_TEXTURE_2D)glRotatef(1, 1, 0, 0) draw_triangle()pygame.display.flip()pygame.time.wait(10)if __name__ == '__main__':main()