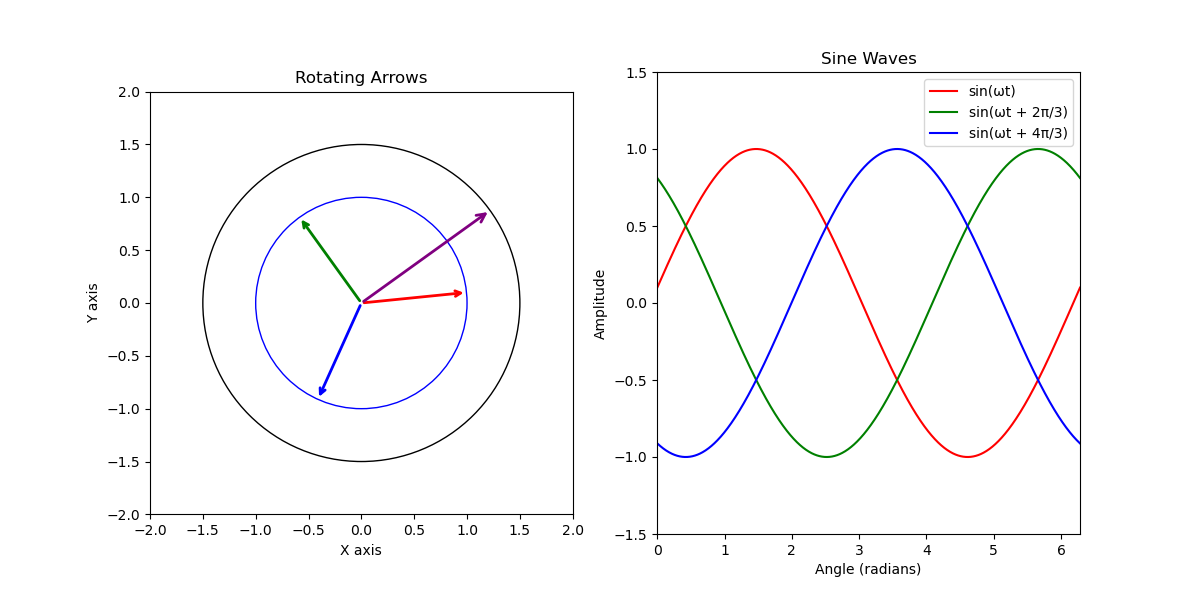
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.patches import Circle, FancyArrowPatch
from matplotlib.animation import FuncAnimation# 创建一个新图和两个坐标轴
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(12, 6))# 设置坐标轴的等比例,确保圆圈是正圆
ax1.set_aspect('equal')
ax2.set_aspect('auto')# 限制坐标轴的范围
ax1.set_xlim(-2, 2)
ax1.set_ylim(-2, 2)
ax2.set_xlim(0, 2 * np.pi)
ax2.set_ylim(-1.5, 1.5)# 设置坐标轴标签
ax1.set_xlabel('X axis')
ax1.set_ylabel('Y axis')
ax2.set_xlabel('Angle (radians)')
ax2.set_ylabel('Amplitude')# 设置标题
ax1.set_title('Rotating Arrows')
ax2.set_title('Sine Waves')# 绘制内外两个圆圈
inner_circle = Circle((0, 0), 1, color='blue', fill=False)
outer_circle = Circle((0, 0), 1.5, color='black', fill=False)
ax1.add_patch(inner_circle)
ax1.add_patch(outer_circle)# 初始化箭头的参数
short_arrow_scale = 1
long_arrow_scale = 1.5
arrow1 = FancyArrowPatch((0, 0), (short_arrow_scale, 0), color='red', mutation_scale=10, arrowstyle='->', lw=2)
arrow2 = FancyArrowPatch((0, 0), (short_arrow_scale, 0), color='green', mutation_scale=10, arrowstyle='->', lw=2)
arrow3 = FancyArrowPatch((0, 0), (short_arrow_scale, 0), color='blue', mutation_scale=10, arrowstyle='->', lw=2)
long_arrow = FancyArrowPatch((0, 0), (long_arrow_scale, 0), color='purple', mutation_scale=15, arrowstyle='->', lw=2)
ax1.add_patch(arrow1)
ax1.add_patch(arrow2)
ax1.add_patch(arrow3)
ax1.add_patch(long_arrow)# 初始化正弦波的线
x_data = np.linspace(0, 2 * np.pi, 1000)
line1, = ax2.plot(x_data, np.sin(x_data), 'r-', label='sin(ωt)')
line2, = ax2.plot(x_data, np.sin(x_data + 2 * np.pi / 3), 'g-', label='sin(ωt + 2π/3)')
line3, = ax2.plot(x_data, np.sin(x_data + 4 * np.pi / 3), 'b-', label='sin(ωt + 4π/3)')# 添加图例
ax2.legend()# 初始化角度
angle = 0# 更新函数,用于动画
def update(frame):global angleangle += 0.05 # 每次旋转0.05弧度if angle > 2 * np.pi: # 如果角度超过2π,则重置角度angle = 0# 更新短箭头的位置arrow1.set_positions((0, 0), (short_arrow_scale * np.cos(angle), short_arrow_scale * np.sin(angle)))arrow2.set_positions((0, 0), (short_arrow_scale * np.cos(angle + 2 * np.pi / 3), short_arrow_scale * np.sin(angle + 2 * np.pi / 3)))arrow3.set_positions((0, 0), (short_arrow_scale * np.cos(angle + 4 * np.pi / 3), short_arrow_scale * np.sin(angle + 4 * np.pi / 3)))# 更新长箭头的位置,错开半个相位long_arrow.set_positions((0, 0), (long_arrow_scale * np.cos(angle + np.pi / 6), long_arrow_scale * np.sin(angle + np.pi / 6)))# 更新正弦波的数据line1.set_ydata(np.sin(x_data + angle))line2.set_ydata(np.sin(x_data + angle + 2 * np.pi / 3))line3.set_ydata(np.sin(x_data + angle + 4 * np.pi / 3))return arrow1, arrow2, arrow3, long_arrow, line1, line2, line3# 创建动画
ani = FuncAnimation(fig, update, frames=np.arange(0, 360), interval=50, blit=True, repeat=True)# 显示图形
plt.show()