一、Xmind整理:
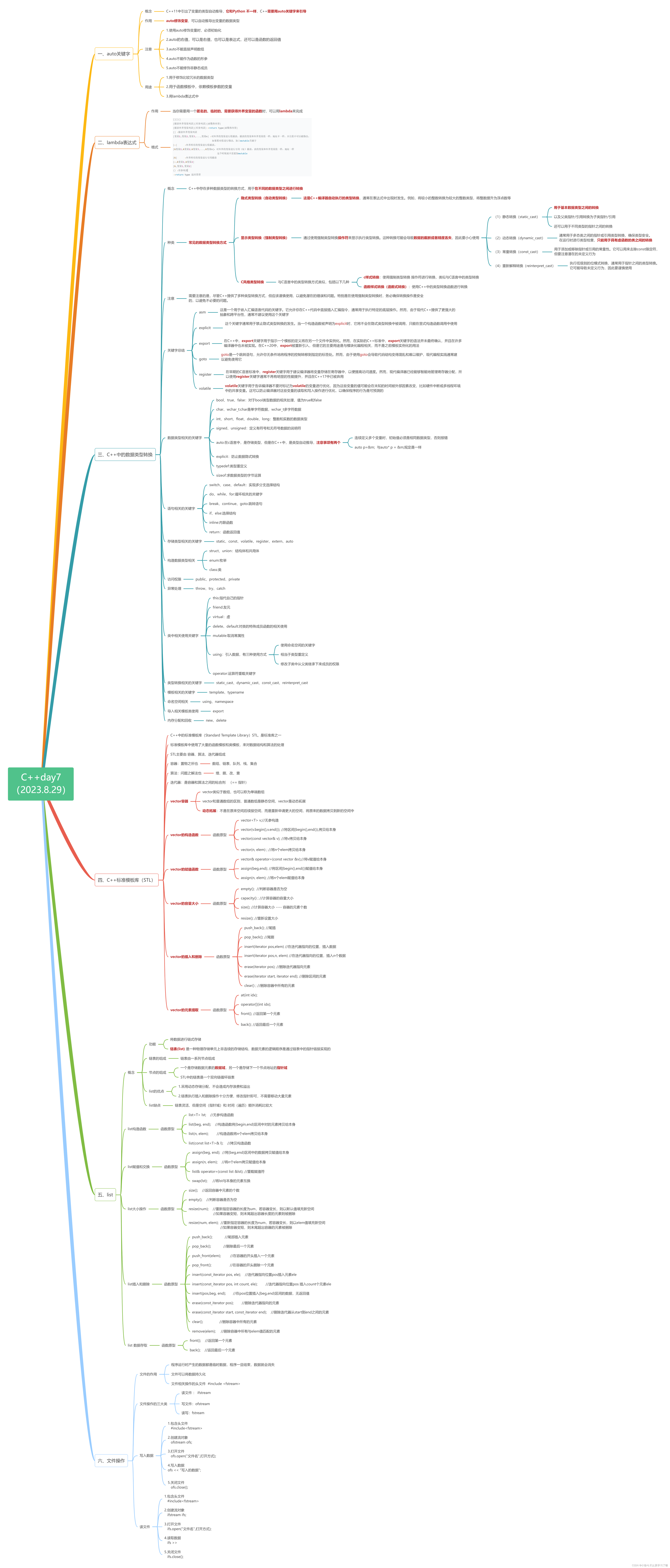
关键词总结:
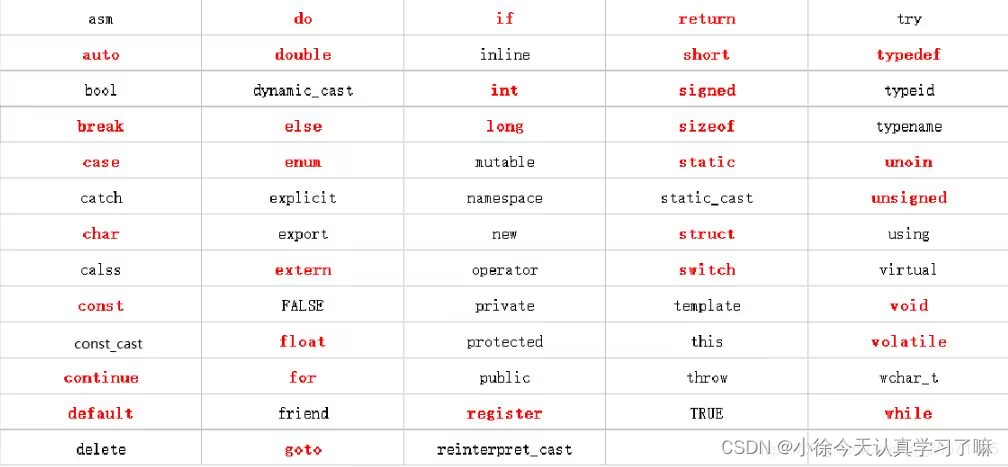
二、上课笔记整理:
1.auto关键字
#include <iostream>using namespace std;int fun(int a, int b, float *c, char d, double *e,int f)
{return 1+2;
}int main()
{//定义一个函数指针,指向fun函数int (*p)(int , int , float *, char , double *,int ) = fun;//用auto来完成auto ptr = fun;return 0;
}
#include <iostream>using namespace std;template <typename T>
void fun(T a)
{auto b = a;cout << "b的数据类型:" << typeid (b).name() << endl;
}int main()
{int a =10;fun(a);return 0;
}
2.lambda表达式
#include <iostream>using namespace std;int main()
{int a = 100;double b = 13.45;char c = 'x';cout << "main-&a:" << &a << " a = " << a << endl;// auto fun = [a,b,c]()mutable{
// auto fun = [=]()mutable{auto fun = [&a,&b](){cout << "lambda--&a " << &a << " a = " << a << endl;a = 200;cout << "lambda--&a " << &a << " a = " << a << endl;};fun();cout << "main-&a:" << &a << " a = " << a << endl;return 0;
}
#include <iostream>using namespace std;int main()
{int a = 100;double b = 13.45;char c = 'x';cout << "main-&a:" << &a << " a = " << a << endl;
// auto fun = [a,b,c]()mutable{
// auto fun = [=]()mutable{auto fun = [&a,&b](string name = "hello world")->string{cout << "lambda--&a " << &a << " a = " << a << endl;a = 200;cout << "lambda--&a " << &a << " a = " << a << endl;return name;};cout << fun("hello kitty") << endl;cout << "main-&a:" << &a << " a = " << a << endl;return 0;
}
3.隐式类型转换(自动类型转换)
int num_int = 10;
double num_double = num_int; // 隐式将int转换为double
4.显示类型转换(强制类型转换)
①静态转换
double num_double = 3.14;
int num_int = static_cast<int>(num_double); // 显式将double转换为int
②动态转换
class Base {virtual void foo() {}
};
class Derived : public Base {};Base* base_ptr = new Derived;
Derived* derived_ptr = dynamic_cast<Derived*>(base_ptr); // 显式将基类指针转换为派生类指针
③常量转换
const int a =10; //
int *p;
p = &a; // 合不合法? no
const int num_const = 5;
int* num_ptr = const_cast<int*>(&num_const); // 去除const限定符
④重新解释转换
int num = 42;
float* float_ptr = reinterpret_cast<float*>(&num); // 重新解释转换
5.C风格类型转换
①c样式转换
int num_int = 10;
double num_double = (double)num_int; // C样式强制类型转换
②函数样式转换(函数式转换)
int num_int = 10;
double num_double = double(num_int); // C++函数样式类型转换
6.vector的构造函数
#include <iostream>
#include <vector>using namespace std;//算法
void printVector(vector<int> &v)
{vector<int>::iterator iter; //定义了这样容器类型的迭代器for(iter = v.begin(); iter != v.end(); iter++){cout << *iter << " ";}cout << endl;
}int main()
{//容器vector<int> v; //无参构造函数v.push_back(10); //尾插v.push_back(20);v.push_back(30);v.push_back(40);//算法printVector(v);vector<int> v2(v.begin(),v.end());printVector(v2);vector<int> v3(6,100);printVector(v3);vector<int> v4 = v3;printVector(v4);vector<int> v5(v2);printVector(v5);return 0;
}
7.vector的容量大小
#include <iostream>
#include <vector>using namespace std;//算法
void printVector(vector<int> &v)
{vector<int>::iterator iter; //定义了这样容器类型的迭代器for(iter = v.begin(); iter != v.end(); iter++){cout << *iter << " ";}cout << endl;
}int main()
{//容器vector<int> v; //无参构造函数v.push_back(10); //尾插v.push_back(20);v.push_back(30);v.push_back(40);//算法printVector(v);vector<int> v2(v.begin(),v.end());printVector(v2);vector<int> v3(6,100);printVector(v3);vector<int> v4 = v3;printVector(v4);vector<int> v5(v2);printVector(v5);vector<int> v6;v6 = v5;printVector(v6);v6.assign(v5.begin(),v5.end());printVector(v6);v6.assign(8,99);printVector(v6);if(v6.empty()){cout << "容器为空" << endl;}else{cout << "容器的容量大小:" << v6.capacity() << endl;cout << "容器的大小:" << v6.size() << endl;v6.resize(15);printVector(v6);}return 0;
}
8.vector的元素提取
#include <iostream>
#include <vector>using namespace std;//算法
void printVector(vector<int> &v)
{vector<int>::iterator iter; //定义了这样容器类型的迭代器for(iter = v.begin(); iter != v.end(); iter++){cout << *iter << " ";}cout << endl;
}int main()
{//容器vector<int> v; //无参构造函数v.push_back(10); //尾插v.push_back(20);v.push_back(30);v.push_back(40);//算法printVector(v);// v.pop_back();//尾删
// printVector(v);// v.insert(v.begin()+1,99);
// printVector(v);// v.insert(v.begin(),4,77);
// printVector(v);// v.erase(v.begin());
// printVector(v);// v.erase(v.begin(),v.end());
// printVector(v);v.clear();printVector(v);cout << "------------------" <<endl;vector<int> vv;for(int i=0;i<5;i++){vv.push_back(i);}cout << vv.at(3) << endl;cout << vv[3] << endl;cout << vv.front() << endl;cout << vv.back() << endl;return 0;
}
9.文件操作
#include <iostream>
#include <fstream>using namespace std;int main()
{//1包含头文件 //2创建流对象ofstream ofs;//3打开文件ofs.open("E:/ready_class/stu.txt",ios::out);//4写入数据ofs << "姓名:张三" << endl;ofs << "年龄:34" << endl;//5关闭文件ofs.close();//1包含头文件//2创建流对象ifstream ifs;//3打开文件ifs.open("E:/ready_class/stu.txt",ios::in);//4读取数据char buff[1024];while(ifs>>buff){cout << buff << endl;}//5.关闭文件ifs.close();return 0;
}