1.请编程实现双向链表的头插,头删、尾插、尾删
2.请编程实现双向链表按任意位置插入、删除、修改、查找
//头文件
#ifndef __HEAD_H__
#define __HEAD_H__
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
typedef char datatype;//定义双向链表结构体
typedef struct Node
{//数据域:存储数据元素datatype data;//指针域:下一个节点的地址struct Node *next;//指针域:上一个节点的地址struct Node *prev;
}*Doublelink;Doublelink inert_head(Doublelink head,datatype element);
Doublelink creat();
void output(Doublelink head);
Doublelink inert_rear(Doublelink head,datatype element);
Doublelink det_head(Doublelink head);
Doublelink det_rear(Doublelink head);
int length(Doublelink head);
Doublelink inert_pos(Doublelink head,int pos,datatype element);
Doublelink det_pos(Doublelink head,int pos);
void find_pos(Doublelink head,int pos);
void cpy_pos(Doublelink head,int pos,datatype element);
#endif
//主函数
#include"head.h"
int main(int argc, const char *argv[])
{Doublelink head=NULL;datatype element;int n;printf("please enter n:");scanf("%d",&n);for(int i=0;i<n;i++){printf("please enter %d element:",i+1);scanf(" %c",&element);// head=inert_head(head,element);head=inert_rear(head,element);}output(head);
//头删
// head=det_head(head);
// output(head);
//尾删
// head=det_rear(head);
// output(head);//任意位置插入int pos;printf("please enter inert pos:");scanf("%d",&pos);printf("please enter inert element:");scanf(" %c",&element);head=inert_pos(head,pos,element);output(head);//任意位置删除printf("please enter det pos:");scanf("%d",&pos);head=det_pos(head,pos);output(head);//查找printf("please enter find pos:");scanf("%d",&pos);find_pos(head,pos);//修改printf("please enter cpy pos:");scanf("%d",&pos);printf("please enter cpy element:");scanf(" %c",&element);cpy_pos(head,pos,element);output(head);return 0;
}
//文本段
#include"head.h"/** function: 创建新节点* @param [ in] * @param [out] * @return 成功返回s 失败返回NULL*/
Doublelink creat()
{Doublelink s=(Doublelink)malloc(sizeof(struct Node));if(s==NULL)return NULL;s->data=0;s->next=s->prev=NULL;return s;
}
/** function: 头插* @param [ in] * @param [out] head,element* @return head*/
Doublelink inert_head(Doublelink head,datatype element)
{Doublelink s=creat();s->data=element;if(NULL==head)head=s;else{s->next=head;head->prev=s;head=s;}return head;
}
/** function: 遍历输出* @param [ in] * @param [out] head* @return head*/
void output(Doublelink head)
{if(NULL==head){puts("empty");return;}else{Doublelink p=head;while(p->next!=NULL)//p到倒数第二个节点{printf("%-5c",p->data);p=p->next;}printf("%-5c\n",p->data);//最后一个元素输出/* while(p)//逆向输出{printf("%-5c",p->data);p=p->prev;}*/// puts("");return;}
}
/** function: 尾插* @param [ in] * @param [out] head,element* @return head*/
Doublelink inert_rear(Doublelink head,datatype element)
{Doublelink s=creat();s->data=element;if(NULL==head)head=s;else{Doublelink p=head;while(p->next!=NULL)//找到最后一个节点{p=p->next;}p->next=s;s->prev=p;}return head;
}
/** function: 头删* @param [ in] * @param [out] head* @return head*/
Doublelink det_head(Doublelink head)
{if(NULL==head)return head;//多个节点>=1Doublelink p=head;head=p->next;head->prev=NULL;free(p);p=NULL;return head;}
/** function: 尾删* @param [ in] * @param [out] head* @return head*/
Doublelink det_rear(Doublelink head)
{if(NULL==head)return head;else if(head->next==NULL)//一个节点{free(head);head=NULL;return head;}else//多个节点>=2{Doublelink p=head;while(p->next)p=p->next;p->prev->next=NULL;free(p);p=NULL;return head;}
}
/** function: 计算链表长度* @param [ in] * @param [out] head* @return len*/
int length(Doublelink head)
{int len=0;while(head)// printf("%-5c\n",p->data);//最后一个元素输出 puts("");{len++;head=head->next;}return len;
}/** function: 任意位置插入* @param [ in] * @param [out] head pos* @return head*/
Doublelink inert_pos(Doublelink head,int pos,datatype element){int len=length(head);Doublelink s=creat();s->data=element;if(NULL==head||pos<1||pos>len+1){puts("error");return NULL; }if(pos==1){head=inert_head(head,element);return head;}Doublelink p=head;for(int i=0;i<pos-1;i++){p=p->next;}Doublelink k=p->prev;k->next=s;s->prev=k;s->next=p;p->prev=s;return head;
}
/** function: 任意位置删除* @param [ in] * @param [out] head pos* @return head*/
Doublelink det_pos(Doublelink head,int pos)
{int len=length(head);//printf("%d\n",len);if(NULL==head||pos<1||pos>len){puts("error");return NULL;}if(pos==1){head=det_head(head);return head;}else if(pos==len){head=det_rear(head);return head;}Doublelink p=head;for(int i=0;i<pos-1;i++)p=p->next;Doublelink k=p->prev;k->next=p->next;p->next->prev=k;free(p);p=NULL;return head;
}
/** function: 逆置* @param [ in] * @param [out] head* @return head*/
Doublelink rever(Doublelink head)
{if(NULL==head)return head;Doublelink p=head->next;head->next=NULL;p->prev=NULL;while(p){Doublelink t=p;p=p->next;t->next=head;head->prev=t;t->prev=NULL;head=t;}return head;
}
void find_pos(Doublelink head,int pos)
{int len=length(head);if(NULL==head||pos<1||pos>len)return ;Doublelink p=head;for(int i=0;i<pos-1;i++)p=p->next;printf("%c\n",p->data);}
void cpy_pos(Doublelink head,int pos,datatype element)
{int len=length(head);if(NULL==head||pos<1||pos>len)return;Doublelink p=head;for(int i=0;i<pos-1;i++)p=p->next;p->data=element;
}
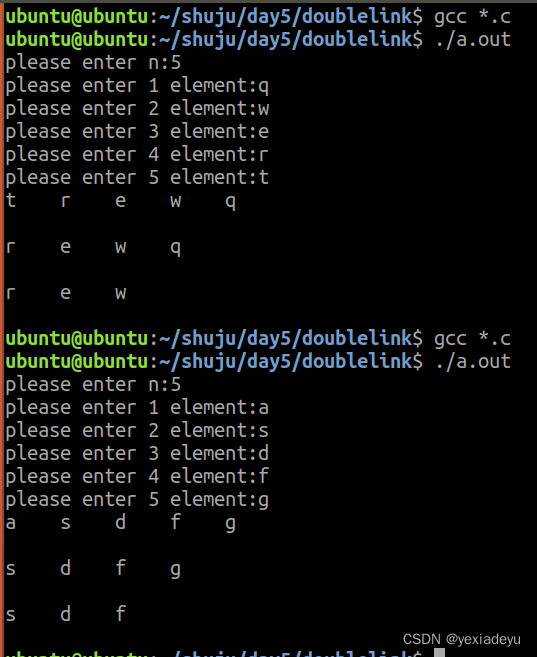
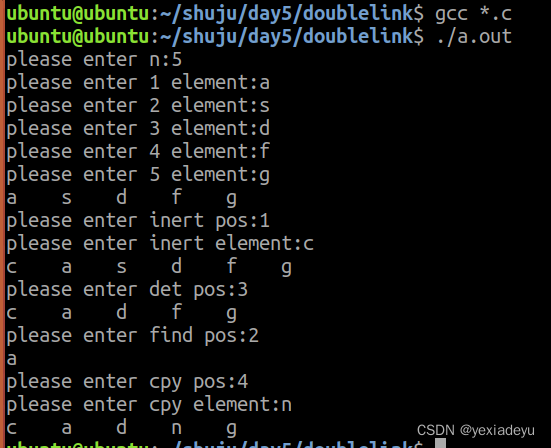
3请简述栈和队列的区别?
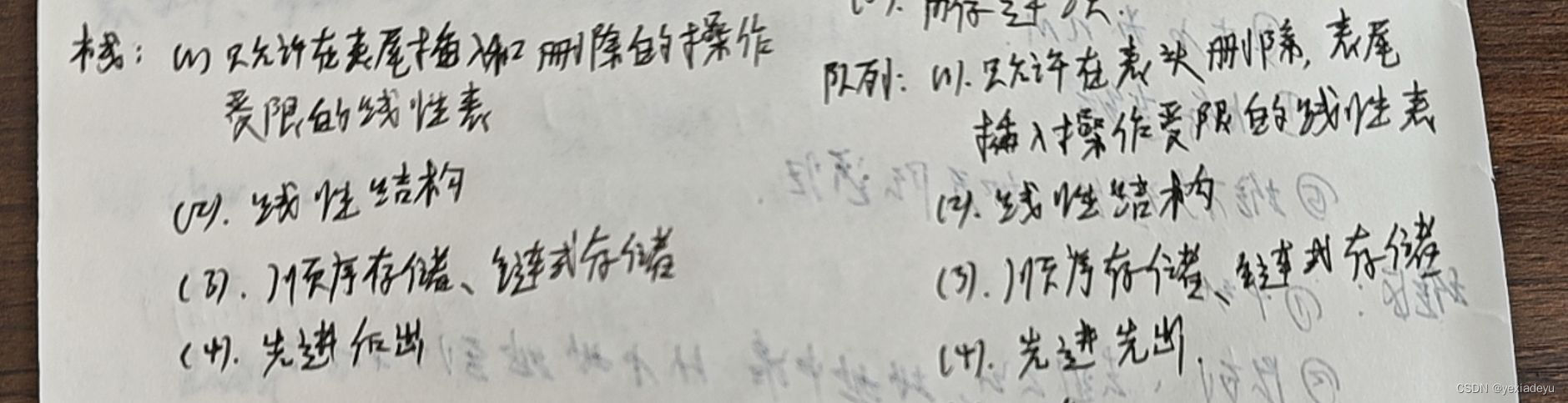
4请简述什么内存泄露?
